JS页面跳转的问题主要可以分为三种情况:跳转到父页面、最外层页面、本页面。下面详细说明每种情况如何进行页面跳转。
JS页面跳转的问题主要可以分为三种情况:跳转到父页面、最外层页面、本页面。下面详细说明每种情况如何进行页面跳转。
跳转到父页面
跳转到父页面时,我们需要使用 window.parent
对象来实现。具体实现方式如下:
// 跳转到父页面
window.parent.location.href = '跳转的目标页面的链接'
示例代码如下:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>父页面</title>
</head>
<body>
<h1>父页面</h1>
<button onclick="jump()">跳转到子页面</button>
<script>
function jump() {
window.location.href = '子页面.html';
}
</script>
</body>
</html>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>子页面</title>
</head>
<body>
<h1>子页面</h1>
<button onclick="jump()">跳转到父页面</button>
<script>
function jump() {
window.parent.location.href = '父页面.html';
}
</script>
</body>
</html>
在子页面中,我们使用 window.parent.location.href
来跳转到父页面。
跳转到最外层页面
跳转到最外层页面时,我们需要使用 window.top
对象来实现。具体实现方式如下:
// 跳转到最外层页面
window.top.location.href = '跳转的目标页面的链接'
示例代码如下:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>最外层页面</title>
</head>
<body>
<h1>最外层页面</h1>
<button onclick="jump()">跳转到子页面</button>
<script>
function jump() {
window.location.href = '子页面.html';
}
</script>
</body>
</html>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>子页面</title>
</head>
<body>
<h1>子页面</h1>
<button onclick="jump()">跳转到最外层页面</button>
<script>
function jump() {
window.top.location.href = '最外层页面.html';
}
</script>
</body>
</html>
在子页面中,我们使用 window.top.location.href
来跳转到最外层页面。
跳转到本页面
跳转到本页面时,我们可以使用 window.location.reload()
来重新加载页面。或者直接改变 location.href
的值来加载跳转到的页面。
示例代码如下:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>本页面</title>
</head>
<body>
<h1>本页面</h1>
<button onclick="jump()">跳转到其他页面</button>
<script>
function jump() {
// 直接跳转到某个页面
window.location.href = '某个页面.html';
// 重新加载当前页面
// window.location.reload();
}
</script>
</body>
</html>
在本页面中,我们可以使用 window.location.href
来直接跳转到其他页面,或者使用 window.location.reload()
来重新加载本页面。
通过上面的三个步骤,我们已经完整地讲解了 JS 页面跳转的问题。
本文标题为:js页面跳转的问题(跳转到父页面、最外层页面、本页面)
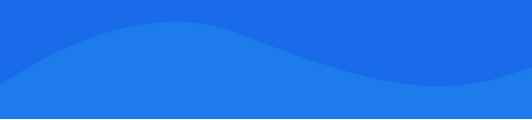
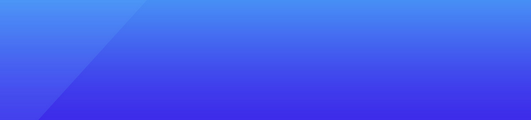
- vue中面包屑的封装 2023-10-08
- javascript 建设银行登陆键盘 2023-11-30
- CSS实现子元素div水平垂直居中的示例 2023-12-14
- HTML - <div>和<span>标签 2023-10-27
- vue 学习小结(3)关于‘Splan‘ 2023-10-08
- html滑动仿悬浮球菜单效果的实现 2022-09-20
- DIV+CSS网页制作布局技巧学习 2023-12-14
- Mysql内储存JSON字符串根据条件进行查询 2024-01-15
- CocosCreator Typescript制作俄罗斯方块游戏 2023-08-08
- ztree+ajax实现文件树下载功能 2023-02-23