本攻略将介绍基于Python实现SQL Server数据库的对象同步。这包括表(Table)、存储过程(Stored Procedure)、触发器(Trigger)等等。通过该攻略,您可以轻松地在不同的数据库之间同步数据,并实现数据库对象的迁移。
基于Python的SQL Server数据库实现对象同步轻量级
本攻略将介绍基于Python实现SQL Server数据库的对象同步。这包括表(Table)、存储过程(Stored Procedure)、触发器(Trigger)等等。通过该攻略,您可以轻松地在不同的数据库之间同步数据,并实现数据库对象的迁移。
需求
在同步数据之前,您需要安装以下软件:
- Python 3.x
- pyodbc 模块
- SQL Server 驱动
您也可以使用pip命令来安装pyodbc模块:
pip install pyodbc
步骤
- 首先,您需要获取源数据库和目标数据库的连接字符串。
import pyodbc
source_conn = pyodbc.connect("DRIVER={SQL Server};SERVER=SourceServerName;DATABASE=SourceDatabaseName;UID=UserID;PWD=Password")
target_conn = pyodbc.connect("DRIVER={SQL Server};SERVER=TargetServerName;DATABASE=TargetDatabaseName;UID=UserID;PWD=Password")
- 然后,您需要实现对象同步的函数。
def sync_objects(source_conn, target_conn, object_type):
cursor_source = source_conn.cursor()
cursor_target = target_conn.cursor()
if object_type == 'tables':
# 获取源数据库中的表的信息
cursor_source.execute("SELECT * FROM sys.tables WHERE type='U'")
tables_source = cursor_source.fetchall()
# 获取目标数据库中的表的信息
cursor_target.execute("SELECT * FROM sys.tables WHERE type='U'")
tables_target = cursor_target.fetchall()
# 创建所有在源数据库中存在的但是在目标数据库中不存在的表
for table_source in tables_source:
if table_source not in tables_target:
cursor_target.execute(f"CREATE TABLE {table_source.tablename}(...)")
print(f"{table_source.tablename} created")
elif object_type == 'stored_procedures':
# 获取源数据库中的存储过程的信息
cursor_source.execute("SELECT * FROM sys.procedures WHERE type='P'")
stored_procedures_source = cursor_source.fetchall()
# 获取目标数据库中的存储过程的信息
cursor_target.execute("SELECT * FROM sys.procedures WHERE type='P'")
stored_procedures_target = cursor_target.fetchall()
# 创建所有在源数据库中存在的但是在目标数据库中不存在的存储过程
for stored_procedure_source in stored_procedures_source:
if stored_procedure_source not in stored_procedures_target:
cursor_target.execute(f"CREATE PROCEDURE {stored_procedure_source.procedurename}(...)")
print(f"{stored_procedure_source.procedurename} created")
elif object_type == 'triggers':
# 获取源数据库中的触发器的信息
cursor_source.execute("SELECT * FROM sys.triggers WHERE type='TR'")
triggers_source = cursor_source.fetchall()
# 获取目标数据库中的触发器的信息
cursor_target.execute("SELECT * FROM sys.triggers WHERE type='TR'")
triggers_target = cursor_target.fetchall()
# 创建所有在源数据库中存在的但是在目标数据库中不存在的触发器
for trigger_source in triggers_source:
if trigger_source not in triggers_target:
cursor_target.execute(f"CREATE TRIGGER {trigger_source.triggername} ON {trigger_source.parentname} ...")
print(f"{trigger_source.triggername} created")
source_conn.close()
target_conn.close()
- 最后,您可以在自己的代码中调用上述函数以完成对象同步。
# 同步源数据库的所有表
sync_objects(source_conn, target_conn, 'tables')
# 同步源数据库的所有存储过程
sync_objects(source_conn, target_conn, 'stored_procedures')
# 同步源数据库的所有触发器
sync_objects(source_conn, target_conn, 'triggers')
示例
示例 1:同步表
假设您需要从名为DatabaseA
的源数据库同步所有表到名为DatabaseB
的目标数据库。
import pyodbc
# 获取源数据库和目标数据库的连接
source_conn = pyodbc.connect("DRIVER={SQL Server};SERVER=SourceServerName;DATABASE=DatabaseA;UID=UserID;PWD=Password")
target_conn = pyodbc.connect("DRIVER={SQL Server};SERVER=TargetServerName;DATABASE=DatabaseB;UID=UserID;PWD=Password")
# 定义同步函数
def sync_objects(source_conn, target_conn, object_type):
cursor_source = source_conn.cursor()
cursor_target = target_conn.cursor()
if object_type == 'tables':
# 获取源数据库中的表的信息
cursor_source.execute("SELECT * FROM sys.tables WHERE type='U'")
tables_source = cursor_source.fetchall()
# 获取目标数据库中的表的信息
cursor_target.execute("SELECT * FROM sys.tables WHERE type='U'")
tables_target = cursor_target.fetchall()
# 创建所有在源数据库中存在的但是在目标数据库中不存在的表
for table_source in tables_source:
if table_source not in tables_target:
cursor_target.execute(f"CREATE TABLE {table_source.tablename}(...)")
print(f"{table_source.tablename} created")
source_conn.close()
target_conn.close()
# 同步所有表
sync_objects(source_conn, target_conn, 'tables')
通过上述代码,您可以轻松地同步名为DatabaseA
的源数据库的所有表到名为DatabaseB
的目标数据库。
示例 2:同步存储过程
假设您需要从名为DatabaseA
的源数据库同步所有存储过程到名为DatabaseB
的目标数据库。
import pyodbc
# 获取源数据库和目标数据库的连接
source_conn = pyodbc.connect("DRIVER={SQL Server};SERVER=SourceServerName;DATABASE=DatabaseA;UID=UserID;PWD=Password")
target_conn = pyodbc.connect("DRIVER={SQL Server};SERVER=TargetServerName;DATABASE=DatabaseB;UID=UserID;PWD=Password")
# 定义同步函数
def sync_objects(source_conn, target_conn, object_type):
cursor_source = source_conn.cursor()
cursor_target = target_conn.cursor()
if object_type == 'stored_procedures':
# 获取源数据库中的存储过程的信息
cursor_source.execute("SELECT * FROM sys.procedures WHERE type='P'")
stored_procedures_source = cursor_source.fetchall()
# 获取目标数据库中的存储过程的信息
cursor_target.execute("SELECT * FROM sys.procedures WHERE type='P'")
stored_procedures_target = cursor_target.fetchall()
# 创建所有在源数据库中存在的但是在目标数据库中不存在的存储过程
for stored_procedure_source in stored_procedures_source:
if stored_procedure_source not in stored_procedures_target:
cursor_target.execute(f"CREATE PROCEDURE {stored_procedure_source.procedurename}(...)")
print(f"{stored_procedure_source.procedurename} created")
source_conn.close()
target_conn.close()
# 同步所有存储过程
sync_objects(source_conn, target_conn, 'stored_procedures')
通过上述代码,您可以轻松地同步名为DatabaseA
的源数据库的所有存储过程到名为DatabaseB
的目标数据库。
本文标题为:基于Python的SQL Server数据库实现对象同步轻量级
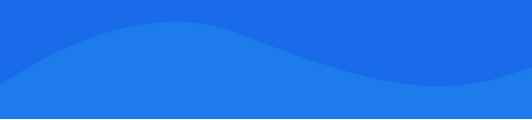
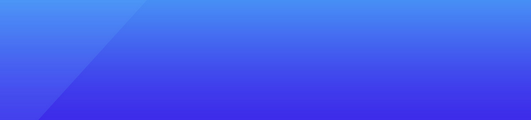
- redis入门介绍及社交行业应用 2023-09-11
- SQL Server中row_number函数用法入门介绍 2023-07-29
- MySQL字段为 NULL的5大坑 2023-07-26
- MySQL数据库索引的弊端及合理使用 2023-08-06
- SpringBoot整合JDBC、Druid数据源的示例代码 2023-12-04
- Redis实现之复制(二) 2023-09-11
- MySQL性能优化 2023-12-03
- Redis实现附近商铺的项目实战 2023-07-12
- springboot整合nacos,如何读取nacos配置文件 2023-12-04
- Oracle DML触发器和DDL触发器实例详解 2023-07-24