How to get IP of all hosts in LAN?(如何获取局域网内所有主机的IP?)
问题描述
我需要列出局域网中所有已连接主机的 IP 地址.最简单的方法是什么?
I need to list IP addresses of all connected hosts in my LAN. What is the simplest way to do this?
推荐答案
您必须执行 ping 扫描.System.Net 命名空间中有一个 Ping 类.示例如下.此外,这只有在您的计算机没有运行防火墙的情况下才有可能.如果他们启用了防火墙,那么除了在您的交换机上执行 SNMP 查询之外,无法确定此信息.
You'll have to do a ping sweep. There's a Ping class in the System.Net namespace. Example follows. Also this is only possible if your computers don't have firewalls running. If they've got a firewall enabled, there's no way to determine this information short of doing SNMP queries on your switches.
System.Net.NetworkInformation.Ping p = new System.Net.NetworkInformation.Ping();
System.Net.NetworkInformation.PingReply rep = p.Send("192.168.1.1");
if (rep.Status == System.Net.NetworkInformation.IPStatus.Success)
{
//host is active
}
另一个问题是确定您的网络有多大.在大多数家庭情况下,您的网络掩码将是 24 位.这意味着它设置为 255.255.255.0.如果您的网关是 192.168.1.1,这意味着您网络上的有效地址将是 192.168.1.1 到 192.168.1.254.这是一个 IP 计算器 来提供帮助.您必须遍历每个地址并使用 Ping 类 ping 地址并检查 PingReply.
The other issue is to determine how large your network is. In most home situations, your network mask will be 24 bits. This means that its set to 255.255.255.0. If your gateway is 192.168.1.1, this means that valid addresses on your network will be 192.168.1.1 to 192.168.1.254. Here's an IP Calculator to help. You'll have to loop through each address and ping the address using the Ping class and check the PingReply.
如果您只是寻找信息而不关心如何获取信息,您可以使用 NMap.命令如下
If you're just looking for the information and aren't concerned with how you get it, you can use NMap. The command would be as follows
nmap -sP 192.168.1.0/24
就速度而言,由于您在本地网络上,因此您可以大大缩短超时间隔,因为您的机器响应时间不应超过 100 毫秒.您还可以使用 SendAsync 并行 ping 它们.以下程序将在半秒内 ping 254 地址.
As far as speed goes, since you're on a local network, you can cut down the timeout interval considerably as your machines shouldn't take more than 100 milliseconds to reply. You can also use SendAsync to ping them all in parallel. The following program will ping 254 address in under half a second.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Net.NetworkInformation;
using System.Diagnostics;
using System.Net;
using System.Threading;
using System.Net.Sockets;
namespace ConsoleApplication1
{
class Program
{
static CountdownEvent countdown;
static int upCount = 0;
static object lockObj = new object();
const bool resolveNames = true;
static void Main(string[] args)
{
countdown = new CountdownEvent(1);
Stopwatch sw = new Stopwatch();
sw.Start();
string ipBase = "10.22.4.";
for (int i = 1; i < 255; i++)
{
string ip = ipBase + i.ToString();
Ping p = new Ping();
p.PingCompleted += new PingCompletedEventHandler(p_PingCompleted);
countdown.AddCount();
p.SendAsync(ip, 100, ip);
}
countdown.Signal();
countdown.Wait();
sw.Stop();
TimeSpan span = new TimeSpan(sw.ElapsedTicks);
Console.WriteLine("Took {0} milliseconds. {1} hosts active.", sw.ElapsedMilliseconds, upCount);
Console.ReadLine();
}
static void p_PingCompleted(object sender, PingCompletedEventArgs e)
{
string ip = (string)e.UserState;
if (e.Reply != null && e.Reply.Status == IPStatus.Success)
{
if (resolveNames)
{
string name;
try
{
IPHostEntry hostEntry = Dns.GetHostEntry(ip);
name = hostEntry.HostName;
}
catch (SocketException ex)
{
name = "?";
}
Console.WriteLine("{0} ({1}) is up: ({2} ms)", ip, name, e.Reply.RoundtripTime);
}
else
{
Console.WriteLine("{0} is up: ({1} ms)", ip, e.Reply.RoundtripTime);
}
lock(lockObj)
{
upCount++;
}
}
else if (e.Reply == null)
{
Console.WriteLine("Pinging {0} failed. (Null Reply object?)", ip);
}
countdown.Signal();
}
}
}
在我自己使用了一些之后,我修改了程序以输出有多少 IP 响应的计数.有一个 const
布尔值,如果设置为 true,将导致程序解析 IP 的主机名.但是,这会显着减慢扫描速度.(不到半秒到16秒)还发现如果IP地址指定错误(自己打错了),回复对象可以为空,所以我处理了.
After some use of it myself, I modified the program to output a count of how many IPs responded. There's a const
bool that if set to true, will cause the program resolve the host names of the IPs. This significantly slows down the scan, though. (under half a second to 16 seconds) Also found that if the IP address is incorrectly specified (made a typo myself), the reply object can be null, so I handled that.
这篇关于如何获取局域网内所有主机的IP?的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:如何获取局域网内所有主机的IP?
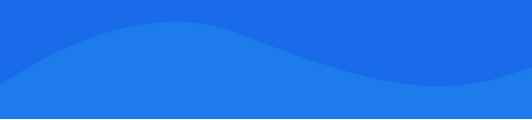
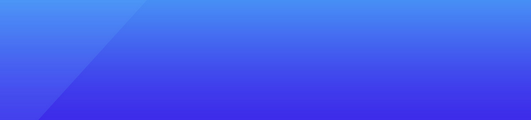
- C# 中多线程网络服务器的模式 2022-01-01
- 带有服务/守护程序应用程序的 Microsoft Graph CSharp SDK 和 OneDrive for Business - 配额方面返回 null 2022-01-01
- Web Api 中的 Swagger .netcore 3.1,使用 swagger UI 设置日期时间格式 2022-01-01
- MoreLinq maxBy vs LINQ max + where 2022-01-01
- 如何用自己压缩一个 IEnumerable 2022-01-01
- WebMatrix WebSecurity PasswordSalt 2022-01-01
- 输入按键事件处理程序 2022-01-01
- C#MongoDB使用Builders查找派生对象 2022-09-04
- 在哪里可以找到使用中的C#/XML文档注释的好例子? 2022-01-01
- 良好实践:如何重用 .csproj 和 .sln 文件来为 CI 创建 2022-01-01